Go back to Blogs
Why is spring boot popular framework in application development?
Spring Boot has become a go to framework for building Java applications, and for good reason. It streamlines the development process significantly, letting developers focus on the core logic of their applications rather than getting bogged down in configuration.
Setting up a traditional Spring application often involves a lot of XML configuration or complex setup. Spring Boot takes an opinionated approach, providing sensible defaults and auto-configuration. This means you can get a production-ready application up and running with minimal effort. It’s like having a pre-configured toolkit ready to go, instead of having to assemble each tool yourself.
Here are a few key reasons why Spring Boot is so popular:
Rapid Development
In software development, time is a critical resource. In a highly competitive market, getting a product to market quickly is essential. Spring Boot simplifies the development process by eliminating boilerplate configuration, allowing developers to focus on business logic rather than setup.
With its auto-configuration and starter dependencies, Spring Boot enables you to set up a project in minutes instead of hours. This streamlined approach significantly reduces development time and effort, helping teams accelerate product delivery. As shown in the figure below, a single click in Spring Initializr is all it takes to start building.
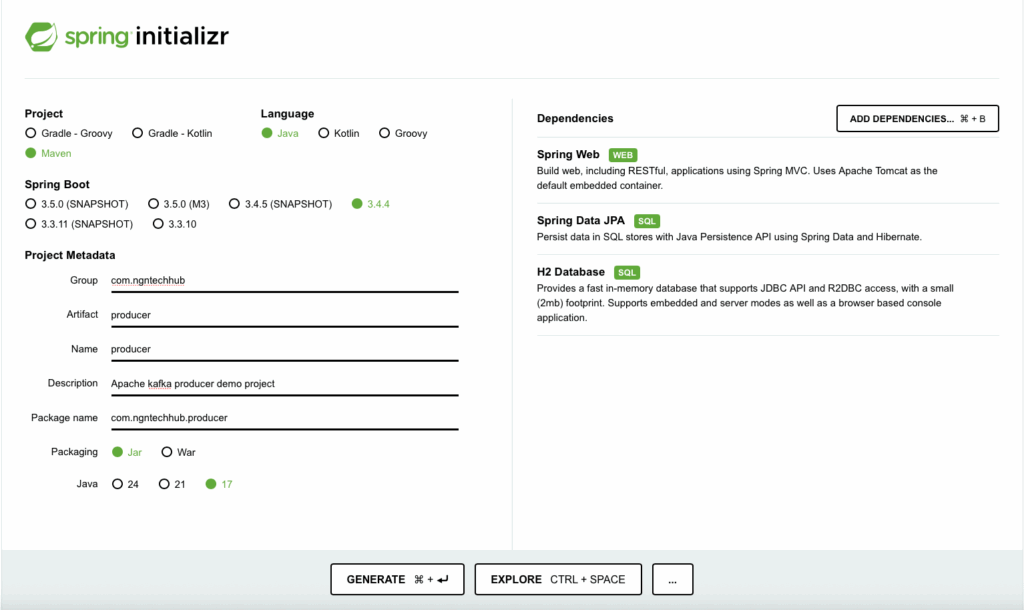
Imagine the advantages of rapid development – delivering faster, gathering stakeholder feedback sooner, and quickly implementing change requests. Spring Boot enables agility and responsiveness, helping you adapt to market demands with ease. By reducing setup time and streamlining development, it allows teams to iterate faster, refine features based on feedback, and stay ahead in a competitive landscape.
Microservice ready
As I’m sure you are aware, microservice architecture is the new age. Even when we design a Mean Valuable Product (MVP) for a small start-up idea, we are thinking in terms of a microservice structure, including asynchronous communication scalability, making it independently deployable, and ensuring flexibility. And guess which framework can help us with that? Yes, Spring Boot!
Regarding the scalability advantages of microservices, we can scale individual components of our application as needed, optimizing resource usage. Spring Boot’s support for building microservices simplifies the process, allowing you to focus on developing the core functionality of each service.
Streamlined configuration
Every developer who has worked on larger or more complex projects will have faced the configuration management nightmare. Traditional approaches usually make a mess of XML files, property files, and environment-specific settings.
Spring Boot follows the “convention over configuration” philosophy, giving sensible defaults, and it provides automatic settings, which reduces the complexity of managing the settings.
Ever imagined a world where you spend less time on the tweaks in configuration files and more on actually writing code? With Spring Boot, simplicity in the configuration will lead to cleaner and more maintainable code. You can do that with Spring Boot by following best practices and avoiding unnecessary boilerplate to focus on the actual functionality of your application.
Please see the following sample XML configuration:
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<!-- Bean Definition -->
<bean id="myBean" class="com.ngntechhub.employee.MyBean">
<property name="propertyName" value="value"/>
</bean>
<!-- Data Source Configuration -->
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/mydb"/>
<property name="username" value="user"/>
<property name="password" value="password"/>
</bean>
</beans>
Introducing a service or bean in XML configuration was complicated and hard to manage, as you can see in the previous XML file. After you write your service, you need to configure it in the XML file as well.
And now we will see how easy it is in Spring Boot. You can write your class with a simple @Service annotation and it becomes a bean:
import org.springframework.stereotype.Service;
@Service
public class MyBean {
private String propertyName = "value";
public String getPropertyName() {
return propertyName;
}
public void setPropertyName(String propertyName) {
this.propertyName = propertyName;
}
}
And the following one is the application properties file. In the previous XML configuration, you saw that it was hard to see and manage data source properties. But in Spring Boot, we can define a data source in a YAML or properties file, as follows:
spring.application.name=employee
spring.datasource.url=jdbc:mysql://localhost:3306/myDb
spring.datasource.username=test
spring.datasource.password=password
spring.datasource.driver-class-name=com.mysql-connector.java
You can see how easy it is to make our code more readable and manageable.
It also promotes collaboration within the development teams through streamlined configuration. When everybody uses the same convention and has the same reliance on the auto-configuration that Spring Boot provides, it reduces the time that would be spent on understanding and working on each other’s code. It means there is consistency in doing things, which promotes efficiency besides minimizing the risk of issues arising from configurations.
Extensive ecosystem
It would be great if we were all just writing code and didn’t require any integrations. But we’re sometimes dealing with complex projects, and all complex projects need a database, messaging between components, and interactions with external services. So, thanks to the Spring ecosystem, we can achieve these by using the libraries and projects of Spring.
As you can see in the figure below, Spring is an ecosystem not just a framework, and each component is ready to communicate with each other smoothly.
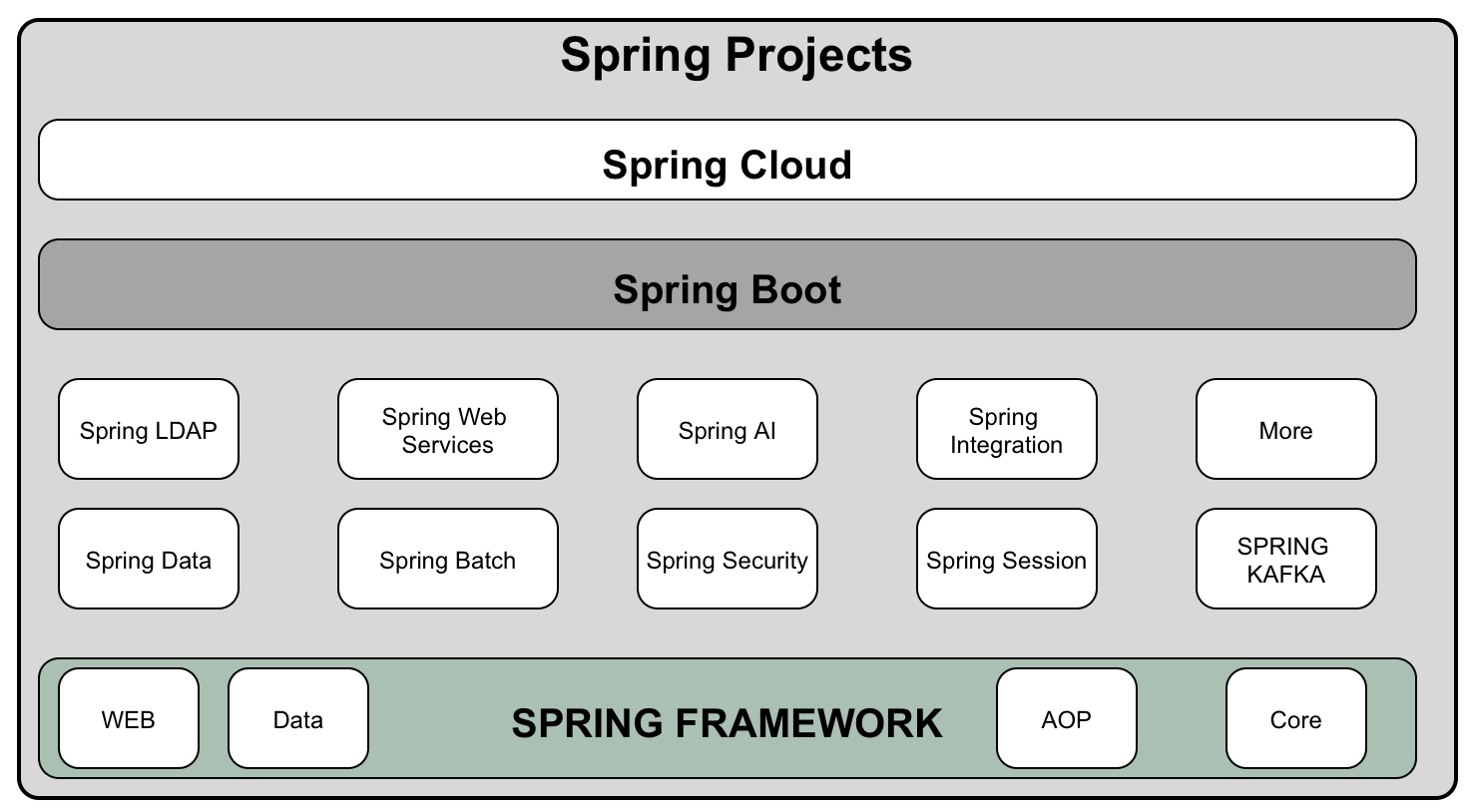
By using the wide ecosystem of Spring Boot, the software development processes can be made quicker, fewer obstacles at various integration levels are encountered, and access to a wide pool of tools and resources is possible. The ecosystem provided by Spring Boot is highly flexible and versatile for enhancing the development process amid the ever-dynamic environment around the development
Cloud-native capabilities
Now, let’s see how Spring Boot best fits into cloud-native development. When we are speaking of cloud-native, in reality, we are referring to applications that are designed for cloud environments such as AWS, Azure, and Google Cloud. Spring Boot has got great features such as scalability and elasticity for applications in such environments, which means our application will grow or shrink horizontally as per demand, plus we get access to multiple managed services.
Testing made easy
Spring Boot really promotes testing and has a lot of tools and conventions to make this possible. That ensures not only saving time in the long run but also a better product for our users. Spring Boot perfectly suits this approach, being all about “testing first.” This approach drives us to consider testing with every step of development, and not as an afterthought.
In a nutshell, Spring Boot equips you with everything that makes your testing efficient and effective. It’s like having a toolkit where each tool is made to address a specific testing need, making your software robust and reliable. Remember that good testing is one of the key elements of quality software development, and Spring Boot stands to guide you through it.
Active development
In software development, keeping up with the times is essential due to the rapid growth of technology. This is where Spring Boot comes into play, being a dynamic framework that is growing with time. In addition, it is actively being developed by a community who are dedicated to adding new features as well as maximizing secure applications. With the help of Spring Boot, you can interact with the latest technology trends, such as newer Java versions or containerization technologies, without starting over each time. This framework continues to change with the industry, to keep your development journey up to date and even closer to the modern progressive foundation upon which your project is built. In the tech world, where everything is constantly changing, Spring Boot works as a handy up-to-date guide empowering you to remain ahead.
Conclusion
The preceding discussion clearly illustrates why Spring Boot has become a cornerstone of modern Java development. Its ability to accelerate development through its “convention over configuration” approach translates directly into significant gains in efficiency and developer productivity. As the demand for rapid software delivery continues to grow, Spring Boot’s streamlined approach will undoubtedly remain a crucial asset for building the complex applications of tomorrow.