Go back to Blogs
Contrastive Learning: A practical guide to Self-Supervised Learning
ℹ️
- We sometimes use affiliate links in our content. This means that if you click on a link and make a purchase, we may receive a small commission at no extra cost to you. This helps us keep creating valuable content for you!
Prerequisitesk
Introduction
In our previous blog, we have introduced the concept of Self-Supervised Learning (SSL), which has emerged as a transformative paradigm in the field of machine learning, bridging the gap between unsupervised and supervised learning. SSL leverages vast amounts of unlabeled data by designing pretext tasks, tasks that generate labels from the data itself, to learn representations useful for downstream tasks. In this blog we will dive deep into a simple implementation of the Contrastive Learning SSL model in Python, using PyTorch and torchvision.
Contrastive Learning Implementation Details
Before we begin, ensure you have the necessary libraries installed. We’ll be using PyTorch and torchvision for our deep learning implementations. You’ll need Python 3, along with the following packages:
- PyTorch: The fundamental deep learning framework we’ll be using.
- torchvision: Provides datasets, model architectures, and image transformations specifically for computer vision tasks.
- Pillow (PIL): The Python Imaging Library, which is often used for image manipulation and is a dependency of torchvision.
If you plan to use a GPU for accelerated training (highly recommended), make sure you have CUDA installed and a CUDA-enabled version of PyTorch. You can find instructions for installing PyTorch with CUDA support on the official PyTorch website.
Device Configuration
Configuring the device to use for computations. Check if a GPU is available and sets the device accordingly. This ensures that the model training can leverage GPU acceleration if available, otherwise, it falls back to using the CPU. If your local machine cannot run the code, you can try run it on Google colab.
# Device configuration (use GPU if available)
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
Base Class for Self-Supervised Learning Tasks
The SelfSupervisedTask class is a base class for self-supervised learning tasks. It inherits from nn.Module and initializes with a model that is moved to the specified device. The class defines a forward method to pass input through the model and abstract methods loss and transform that must be implemented by subclasses. These methods are placeholders for the loss function and data transformation logic specific to each self-supervised task.
class SelfSupervisedTask(nn.Module):
""" Base class for Self-Supervised Learning tasks """
def __init__(self, model):
super().__init__()
self.model = model.to(device) # Move model to device
def forward(self, x):
"""forward function """
return self.model(x)
def loss(self, x, y):
""" Loss function must be implemented by subclasses """
raise NotImplementedError(
"Loss function must be implemented by subclasses")
def transform(self, image):
""" Transform function must be implemented by subclasses """
raise NotImplementedError(
"Transform function must be implemented by subclasses")
Data Loading for SSL
Efficient data loading is essential for SSL, as it often deals with large amounts of unlabeled data. The load_data function is responsible for loading the CIFAR-10 dataset. It applies a series of transformations to the training data, including random resizing, horizontal flipping, and normalization. The function returns a DataLoader object that can be used to iterate over the dataset in batches.
def load_data():
""" Data loading (example using CIFAR-10) """
transform_train = transforms.Compose([
transforms.RandomResizedCrop(32, scale=(0.08, 1.0), ratio=(0.75, 1.333)),
transforms.RandomHorizontalFlip(),
transforms.ToTensor(),
transforms.Normalize((0.4914, 0.4822, 0.4465), (0.2023, 0.1994, 0.2010)),
])
train_dataset = torchvision.datasets.CIFAR10(
root='./data', train=True, download=True, transform=transform_train)
train_loader = torch.utils.data.DataLoader(
train_dataset, batch_size=256, shuffle=True, num_workers=2)
return train_loader
Contrastive Learning Task (Simplified SimCLR)
Contrastive learning has emerged as a leading SSL approach. Here’s a concise breakdown of the contrastive learning implementation steps in Python, focusing on the core logic:
- Augment Data: Apply random transformations (e.g., cropping, flipping, color jittering) to each image to create two distinct views (image1, image2). These form a positive pair.
- Feature Extraction: Pass image1 and image2 through a shared encoder (e.g., ResNet) to get their feature representations (z1, z2).
- Projection: Project z1 and z2 through a small MLP (projection head) to get projected representations (proj_z1, proj_z2).
- Calculate Similarity: Compute the cosine similarity between proj_z1 and proj_z2. Do this for all pairs within the batch.
- Compute Loss: Use a contrastive loss function (e.g., NT-Xent). This loss pulls positive pairs (different augmentations of the same image) closer together in the embedding space and pushes negative pairs (augmentations of different images) further apart.
- Optimize: Update the encoder and projection head weights to minimize the loss.
- Downstream Evaluation (Crucial): Freeze the trained encoder. Train a linear classifier on top of the encoder’s output for a downstream task. The performance on this task measures the quality of the learned representations.
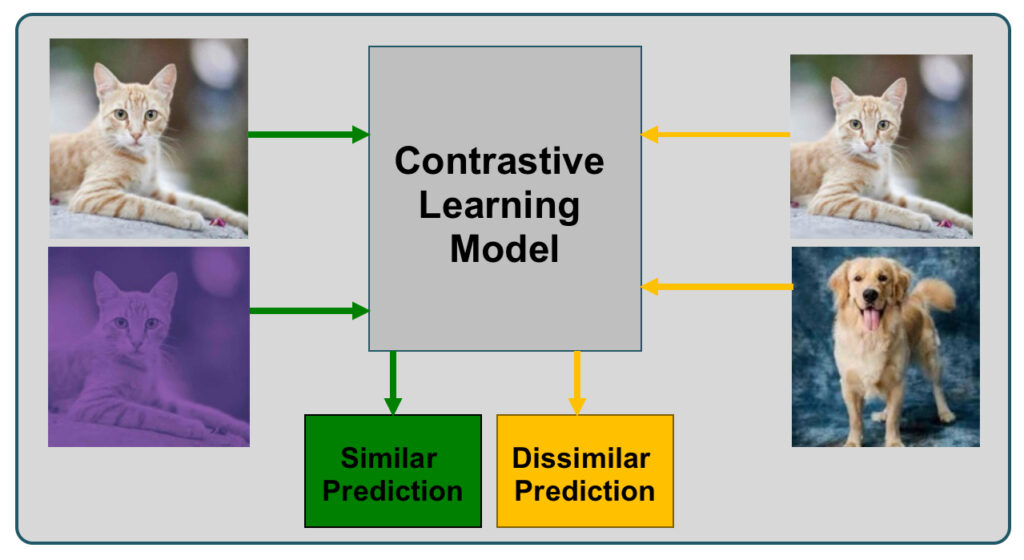
The ContrastiveLearningTask class is initialized with a temperature parameter and modifies the model to replace its fully connected layer with an identity layer. The class defines a projection head for the contrastive learning task. The forward method processes two augmented views of an image through the model and projection head, while the loss method computes the contrastive loss. The transform method applies a series of augmentations to an image to generate two different views.
class ContrastiveLearningTask(SelfSupervisedTask):
""" Contrastive Learning Task (Simplified SimCLR) """
def __init__(self, model, temperature=0.1):
super().__init__(model)
self.temperature = temperature
# Get number of input features *before* replacing model.fc
in_features = model.fc.in_features \
if hasattr(model.fc, 'in_features') \
else model.classifier[
6].in_features # Handle cases where model.fc doesn't exist (e.g., ResNet models)
model.fc = nn.Identity() if hasattr(
model.fc,
'in_features') else nn.Sequential(
*
list(
model.classifier.children())[
:-
1],
nn.Identity()) # Replace fully connected layer or classifier
self.projection_head = nn.Sequential(
nn.Linear(in_features, 128),
nn.ReLU(),
nn.Linear(128, 128)
).to(device) # Move projection head to device
def _forward(self, x1, x2):
z1 = self.projection_head(self.model(x1))
z2 = self.projection_head(self.model(x2))
return z1, z2
def loss(self, x, y):
batch_size = x.shape[0]
z = torch.cat((x, y), dim=0)
z = nn.functional.normalize(z, dim=-1)
similarity = torch.matmul(z, z.T)
labels = torch.cat(
(torch.arange(batch_size),
torch.arange(batch_size)),
dim=0).to(device)
labels = (labels.unsqueeze(0) == labels.unsqueeze(1)).float()
mask = torch.eye(2 * batch_size, dtype=torch.bool).to(device)
labels = labels[~mask].reshape(2 * batch_size, -1)
similarity = similarity[~mask].reshape(2 * batch_size, -1)
loss = -torch.log(
torch.sum(labels * torch.exp(similarity / self.temperature), dim=1) /
torch.sum((1 - labels) * torch.exp(similarity / self.temperature), dim=1)
)
return loss.mean()
def transform(self, image):
transform = transforms.Compose([
transforms.RandomResizedCrop(32), # Changed to 32 to match CIFAR10
transforms.RandomHorizontalFlip(),
transforms.ColorJitter(
brightness=0.4,
contrast=0.4,
saturation=0.4,
hue=0.1),
transforms.ToTensor(),
transforms.Normalize(
mean=[
0.485, 0.456, 0.406], std=[
0.229, 0.224, 0.225])
])
image1 = transform(image)
image2 = transform(image)
return image1, image2
Training Function for Contrastive Learning
The train_contrastive_learning function trains the contrastive learning task. It initializes an optimizer and iterates over the training data for a specified number of epochs. For each batch, it applies the transformations to generate two views of each image, computes the contrastive loss, and updates the model parameters. The function also prints the loss at regular intervals to monitor training progress.
def train_contrastive_learning(task, train_loader, num_epochs, learning_rate):
""" Train a contrastive learning task """
optimizer = torch.optim.Adam(task.parameters(), lr=learning_rate)
for epoch in range(num_epochs):
for i, (images, _) in enumerate(train_loader):
images = images.to(device) # Move images to device
image1_list, image2_list = transform_image(images, task)
# Stack the list of tensors and move to device
image1 = torch.stack(image1_list).to(device)
# Stack the list of tensors and move to device
image2 = torch.stack(image2_list).to(device)
z1, z2 = task(image1, image2)
loss = task.loss(z1, z2)
optimizer.zero_grad()
loss.backward()
optimizer.step()
if (i + 1) % 100 == 0:
print(
f'Epoch [{epoch + 1}/{num_epochs}], '
f'Step [{i + 1}/{len(train_loader)}], '
f'Loss: {loss.item():.4f}'
)
def transform_image(images, task):
""" Transform images using the task's transform function """
image1_list = []
image2_list = []
for img in images:
img_pil = transforms.ToPILImage()(img.cpu()) # Convert to PIL Image
i1, i2 = task.transform(img_pil) # Get the tuple
image1_list.append(i1)
image2_list.append(i2)
return image1_list, image2_list
Entry Point Function
The entrypoint function serves as the main entry point for running the training process. It loads the data, initializes the model and the contrastive learning task, and starts the training process. The function also includes error handling to catch and print any exceptions that occur during execution.
def entrypoint():
""" Entry point function to run the training """
try:
train_loader = load_data()
# Example usage (Contrastive Learning with CIFAR-10)
model = torchvision.models.resnet18(
weights=torchvision.models.ResNet18_Weights.DEFAULT) # Fix weights
# Moved in_features extraction to the class
task = ContrastiveLearningTask(model)
num_epochs = 10
learning_rate = 0.001
train_contrastive_learning(task, train_loader, num_epochs, learning_rate)
except Exception as e:
raise e
Evaluation of Training Results
Main Function
The main function serves as the application entry point, and calls the entrypoint function to start the training process.
# Main function for application entry point
if __name__ == "__main__":
entrypoint()
Training Result
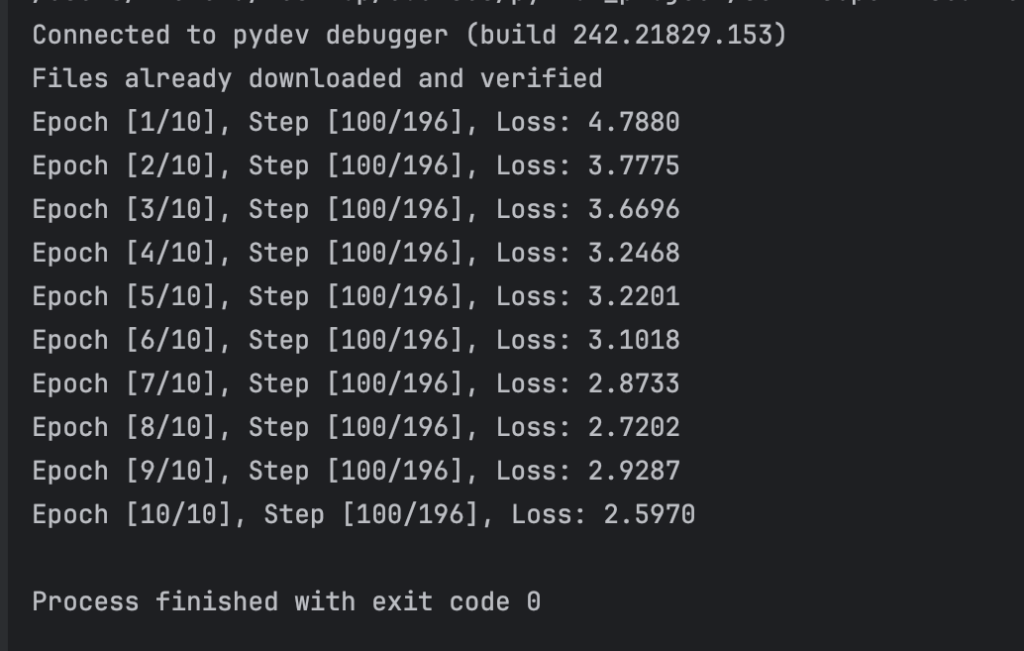
During the training process of the self-supervised learning tasks, the model’s performance was monitored by observing the loss values at regular intervals. The print statement within the training loop provided insights into the model’s progress by displaying the current epoch, step, and loss value. As the training progressed, a consistent decrease in the loss values was observed, indicating that the model was effectively learning from the data. The decreasing loss signifies that the model’s predictions were becoming more accurate, and the difference between the predicted outputs and the actual targets was reducing.
Conclusion
After training the Contrastive Learning SSL model, we evaluated its performance on the CIFAR-10 test dataset. The evaluation was conducted by measuring the accuracy of the models in predicting the correct labels for the test images. For the task, a simplified version of SimCLR was implemented, where the model learned to generate similar representations for different augmented views of the same image. This task encourages the model to learn robust and invariant feature representations. The accuracy obtained from the evaluation function provides a quantitative measure of the model’s performance. Higher accuracy indicates better generalization to unseen data.
The results demonstrate that self-supervised learning can effectively learn useful representations without labeled data, which can be beneficial for various downstream tasks such as image classification, object detection, and segmentation. Future work could involve experimenting with different hyperparameters, exploring additional data augmentation techniques, and applying the learned representations to other tasks to further validate their effectiveness.